When developing an Android application, developers must first choose which database to use, especially if the mobile application is database intensive.
When developers start looking for the best database, they can find many different solutions, but when data needs to be saved on a user’s device, there are two major options that will come in handy. There are Realm and SQLite.
SQLite is one of the most popular and easy-to-use database management systems for this purpose. Although SQLite is widely used, it has some limitations. Structured Query Language (SQL) requests may be slow, and it may be difficult to manage a huge set of data. It’s also harder to migrate a program as the amount of data increases. Realm is an easy-to-use, open-source alternative to SQLite. The key difference between the two is that Realm is an object database management system, while SQLite is a relational database management system. Realm is often used to replace SQLite.
Let’s take a closer look at both databases.
What is SQLite?
SQLite, designed in 2000, is a C-language library that implements a small, fast, autonomous, highly reliable, and full-featured SQL database engine. SQLite is a relational database management system. The data is stored in the form of relational tables, which consist of rows and columns. Tables can be interrelated, and their columns can be merged if necessary. SQLite uses queries, and the results of the queries are compared with objects. Changing a database, for example, adding columns, may require schema migration. SQLite is also portable and supports a variety of third-party libraries. It may be difficult to manage a complex database as you may need to write SQL commands. “Lite,” as part of SQLite’s name, refers to it being a lightweight database in terms of resource usage, database administration, and setup.
What is Realm?
The Realm database is an open-source, easy-to-use alternative to Core Data and SQLite. It’s a non-relational, NoSQL database that allows you to set up relationships between different objects. Realm is far younger than SQLite; nonetheless, it’s widely popular among mobile developers around the globe. This tool is described as a database management system that can easily replace SQLite. First called TightDB, its development began in 2010. In 2014, the developers renamed the tool to Realm and launched its beta version. The database, developed from scratch, operates on a custom storage engine. Realm is widely used for mobile app development, especially among junior Android developers. This database flawlessly works with Java, Kotlin, Swift, Objective-C, Xamarin, and React Native.
The main advantage of Realm, in contrast to SQLite, is enhanced speed and effectiveness. It’s user-friendly and cross-platform. However, a new library for SQLite, Room, was introduced in 2017 and became a turning point in the competition between Realm and SQLite.
Room
Today, it’s not easy to find a developer who codes a mobile app with SQLite alone. Here, Room comes in handy.
Room, which you can learn more about here, is a high-level interface for low-level SQLite bindings embedded in Android. Room does the major part of its work during compilation, creating an API interface over embedded SQLite API. As a result, you don’t need to work with Cursor or ContentResolver.
Let's compare Room and SQLite vs. Realm.
Size
Realm is a far bigger library than Room because it includes a separate database. It adds around 3-4 MB to your app’s APK. Since Room is merely a layer over SQLite, which is built into the OS, it adds only a couple of dozen KB to your APK. Room also includes far fewer methods.
Multithreading
Realm requires that objects are not transferred between streams. Data objects in Realm are values that change according to the changes made in a database. That’s why they are connected to a given stream where a Realm instance exists and from which they were received (if this Realm instance is closed, the extracted objects become invalid). In my experience, this usually isn’t a big problem if you are careful enough. But if you switch streams, you will have to create new Realm instances and re-run queries to extract your objects. Room has no such stream restrictions.
Security
Room stores its SQLite file in an inner data catalog of an app. So, no other application can access it, including a user, if the smartphone isn’t rooted. This is a good thing for common use cases. If you need an extra security layer, you can use SQLCipher to encrypt your database. You can use SQLCipher directly with Room.
SQLCipher is an SQLite extension that allows the use of 256-bit encryption of SQLite databases. You can use the SQLCipher namespace instead of SQLite API interfaces for objects with similar names to facilitate migration.
Realm encrypts and decodes mobile app data transparently, with standard AES-256 encryption, using the first 256 bits of the provided 512-bit encryption key. The remaining 256 bits are used to check integrity with HMAC (Hash-based Message Authentication Code).
Speed
Below there are several graphs comparing the speed of executing the CRUD operations on various datasets for SQLite, Room, and Realm:
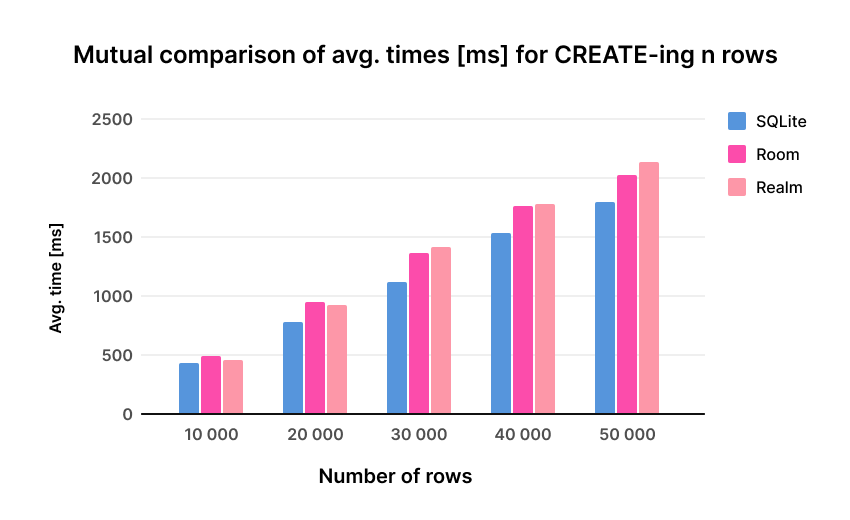
Rank: SQLite, Room, Realm.
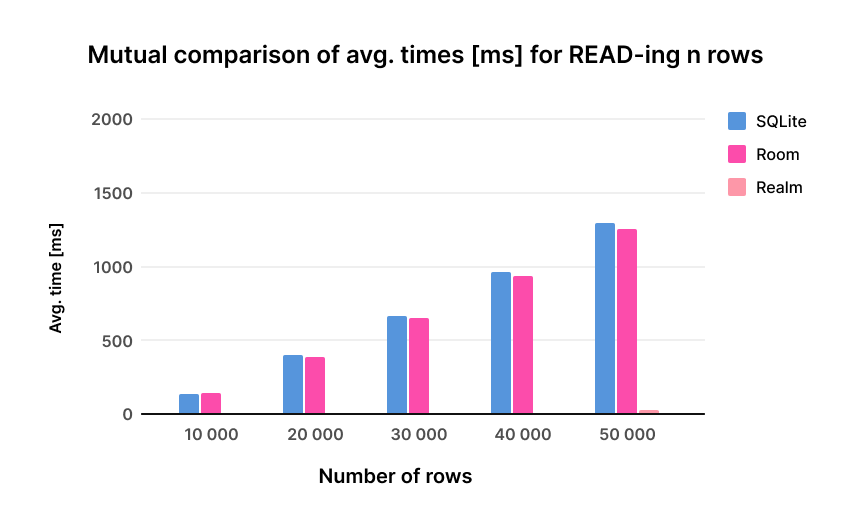
Rank: Realm, Room, SQLite.
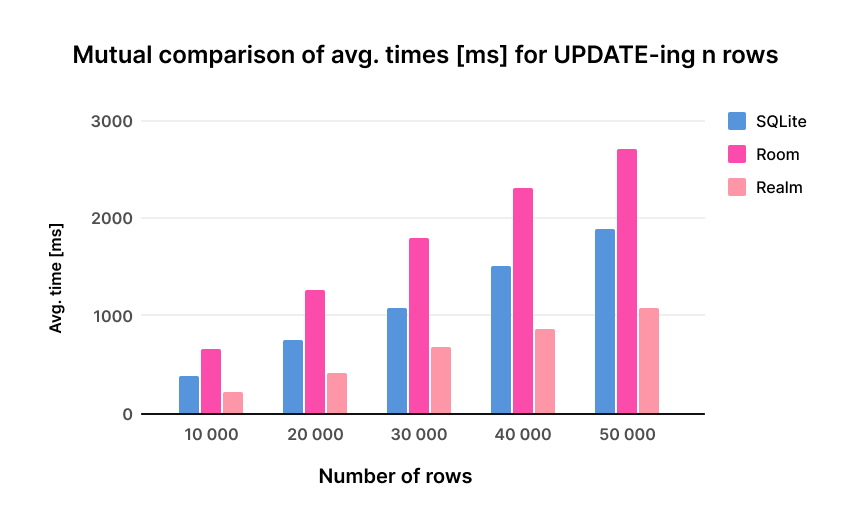
Rank: Realm, SQLite, Room.
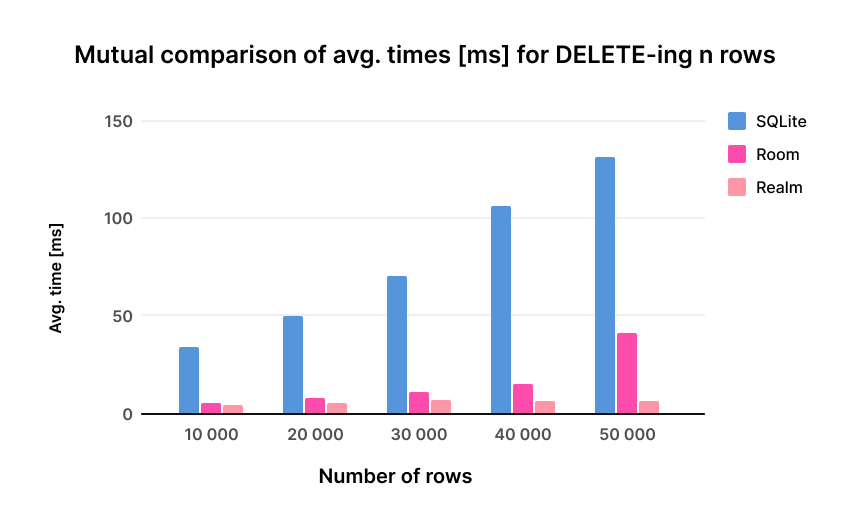
Rank: Realm, Room, SQLite.
Thus, the tests show that Realm is faster than SQLite and Room. You can learn more about this performance comparison here.
Limitations of Realm
Realm has a number of limitations:
1. Realm does not have support for auto-incrementing ID’s and composite keys.
2. There is no support for nested transactions.
3. Using Realm, we can’t request to merge tables. For example:
Select user.name AS userName, pet.name AS petName FROM user, pet WHERE user.id = pet.user_id
So, it’s difficult to make requests consisting of 4–5 tables in Realm.
4. Moreover, in Realm, if we want to create a POJO (plain old Java object), we need to do it manually, while Room allows us to return a value expression.
Summing up
SQLite database may scare away a junior developer because of its complexity. That’s why the majority of junior software engineers use Realm in their first Android projects. But when the projects get more complex and large-scale, you will realize that SQLite coupled with Room is the best option for you. Realm can be a good choice if you have an application with a very simple database. But in practice, this does not happen. When the application starts to expand, and manipulations in the database become more serious than just putting and getting data from the table, you will understand the pain of NoSQL databases.
Also, you shouldn’t forget about Google’s support. Room is included in Android Jetpack and, together with other Jetpack libraries, allows you to build an Android app correctly, with minimum glitches and memory leaks.